Internet Java Engineer Advanced Knowledge Complete Literacy
Most of the content of this project comes from Chinese Hushan, and the copyright belongs to the author. The content covers knowledge in the fields of high concurrency, distribution, high availability, microservices, and massive data processing. We have systematically organized this part of knowledge to facilitate readers' learning and reference.
We are also working hard to update the algorithm project! If you are preparing for written interview algorithms, or want to further improve your coding skills, you are welcome to Star and follow doocs/leetcode
Before studying this project, let's take a look at what the technical interviewers in the Discussions forum said. This project welcomes all developer friends to share some of their ideas and practical experiences in the Discussions forum. You may also wish to follow doocs/advanced-java with Star to keep track of the latest developments in the project.
High concurrency architecture
message queue
- Why use message queue? What are the advantages and disadvantages of message queues? What are the advantages and disadvantages of Kafka, ActiveMQ, RabbitMQ, and RocketMQ?
- How to ensure the high availability of message queue?
- How to ensure that messages are not consumed repeatedly? (How to ensure the idempotence of message consumption)
- How to ensure the reliable transmission of messages? (How to deal with the problem of message loss)
- How to ensure the order of messages?
- How to solve the message queue delay and expiration problems? What to do when the message queue is full? There are millions of messages that have been backlogged for several hours. How can we solve them?
- If you were asked to write a message queue, how would you design the architecture? Tell me your thoughts.
search engine
- Can you explain the principle of distributed architecture of ES (how does ES achieve distribution)?
- How does ES write data work? How does ES query data work? Can you introduce the underlying Lucene? Do you know about inverted index?
- How can ES improve query efficiency when the amount of data is large (billions of levels)?
- What is the deployment architecture of ES production cluster? Approximately how much data does each index have? Approximately how many shards are there per index?
cache
- How is caching used in the project? What are the consequences of caching if used incorrectly?
- What is the difference between Redis and Memcached? What is the threading model of Redis? Why is single-threaded Redis much more efficient than multi-threaded Memcached?
- What data types does Redis have? In which scenarios is it more suitable to use?
- What are the expiration strategies of Redis? Can you write the LRU code by hand?
- How to ensure high concurrency and high availability of Redis? Can you introduce the master-slave replication principle of Redis? Can you introduce the sentinel principle of Redis?
- What is the master-slave architecture of Redis?
- How does Redis Sentinel Cluster achieve high availability?
- What are the persistence methods of Redis? What are the advantages and disadvantages of different persistence mechanisms? How is the underlying persistence mechanism implemented?
- Can you explain how Redis cluster mode works? In cluster mode, how are Redis keys addressed? What are the algorithms for distributed addressing? Do you understand the consistent hash algorithm? How to dynamically add and delete a node?
- Understand what is Redis avalanche, penetration and breakdown? What happens after Redis crashes? How should the system deal with this situation? How to deal with Redis penetration?
- How to ensure double-write consistency between cache and database?
- What is the concurrency contention issue in Redis? How to solve this problem? Do you know the CAS solution for Redis transactions?
- How is Redis deployed in the production environment?
- Have you ever understood the process of Redis rehash?
Sub-database and sub-table
- Why should we divide databases and tables (when designing a high-concurrency system, how should the database level be designed)? Which database and table sharding middleware have you used? What are the advantages and disadvantages of different sub-database and sub-table middleware? How do you split the database vertically or horizontally?
- Currently, there is a system that is not divided into databases and tables. In the future, it will be divided into databases and tables. How to design it so that the system can dynamically switch from undivided databases and tables to divided databases and tables?
- How to design a sub-database and table scheme that can dynamically expand and shrink?
- After the database is divided into tables, how to deal with the id primary key?
Read and write separation
- How to realize the separation of reading and writing in MySQL? What is the principle of MySQL master-slave replication? How to solve the delay problem of MySQL master-slave synchronization?
High concurrency system
- How to design a high-concurrency system?
distributed system
Interview serial bombardment
System split
- Why system split? How to perform system split? Is it okay not to use Dubbo after splitting?
Distributed service framework
- Tell me how Dubbo works? Can communication continue if the registration center is down?
- What serialization protocols does Dubbo support? Tell me about the data structure of Hessian? Does PB know? Why is PB the most efficient?
- What are Dubbo's load balancing strategies and cluster fault tolerance strategies? What about dynamic proxy strategies?
- What is Dubbo's spi idea?
- How to perform service governance, service degradation, failure retry and timeout retry based on Dubbo?
- How to design the idempotence of distributed service interfaces (for example, no repeated deductions)?
- How to ensure the order of distributed service interface requests?
- How to design an RPC framework similar to Dubbo yourself?
- What is P of the CAP theorem?
Distributed lock
- What are the application scenarios of Zookeeper?
- How to design distributed locks using Redis? Is it possible to use Zookeeper to design distributed locks? Which of the above two distributed lock implementation methods is more efficient?
Distributed transactions
- Do you understand distributed transactions? How do you solve the distributed transaction problem? TCC What should I do if the network fails to connect? How to ensure the consistency of XA?
distributed session
- How to implement distributed Session during cluster deployment?
Highly available architecture
- Introduction to Hystrix
- E-commerce website details page system architecture
- Hystrix thread pool technology implements resource isolation
- Hystrix semaphore mechanism implements resource isolation
- Fine-grained control of Hystrix isolation policies
- Deep dive into Hystrix execution internals
- Optimize batch product data query interface based on request cache request caching technology
- Fallback degradation mechanism based on local cache
- An in-depth look at the operating principles of Hystrix circuit breakers
- Deep dive into Hystrix thread pool isolation and interface current limiting
- Provide security protection for service interface call timeout based on timeout mechanism
Highly available system
- How to design a highly available system?
Current limiting
- How to limit the flow? How do you do it at work? Tell me about the specific implementation?
fuse
- How to perform circuit breaker?
- What are the fuse frameworks? Do you know the specific implementation principle?
- How to make technical selection of fuse frame? Sentinel or Hystrix?
Downgrade
Microservice architecture
- The entire chapter on microservice architecture is an additional addition and will be updated later. Readers are also welcome to participate in supplementary improvements.
- Description of microservice architecture
- Migrate from monolithic architecture to microservices architecture
- Event-driven data management for microservices
- Choose a microservice deployment strategy
- Advantages and disadvantages of microservice architecture
Spring Cloud microservice architecture
- What are microservices? How do microservices communicate independently?
- What are the differences between Spring Cloud and Dubbo?
- Spring Boot and Spring Cloud, talk about your understanding of them?
- What is a service circuit breaker? What is service downgrade?
- What are the advantages and disadvantages of microservices? Tell us about the pitfalls you encountered during project development?
- What microservice technology stacks do you know?
- Microservice governance strategy
- Both Eureka and Zookeeper can provide service registration and discovery functions. What is the difference between them?
- Let’s talk about the main calling process of the service discovery component Eureka?
- ...
Massive data processing
- How to find the same URL from a large number of URLs?
- How to find high-frequency words from large amounts of data?
- How to find out the IP that visits Baidu website the most on a certain day?
- How to find unique integers in a large amount of data?
- How to determine whether a number exists in a large amount of data?
- How to query the most popular query strings?
- How to count the number of different phone numbers?
- How to find the median from 500 million numbers?
- How to sort by query frequency?
- How to find the top 500 numbers?
- Tell me about the common routines for TopK problems in big data?
Stars Trends
Note: This trend chart is automatically refreshed regularly by actions-starcharts, author @MaoLongLong
Doocs community quality projects
The Doocs technology community is committed to creating a complete and continuously growing learning ecosystem for Internet developers! The following are some excellent projects under Doocs. Developer friends are welcome to continue to pay attention.
# | project | describe | heat |
---|
1 | advanced-java | Internet Java engineers are completely literate in advanced knowledge: covering knowledge in areas such as high concurrency, distribution, high availability, microservices, and massive data processing. |
|
2 | leetcode | A variety of programming languages implement LeetCode, "Sword Pointer Offer (2nd Edition)", "Programmer Interview Code (6th Edition)" problem solutions. |
|
3 | source-code-hunter | Source code analysis of common Internet component frameworks. |
|
4 | jvm | Summary of knowledge on the underlying principles of Java virtual machine. |
|
5 | coding-interview | A collection of coding interview questions, including "The Sword Points to Offer", "The Beauty of Programming", etc. |
|
6 | md | A highly concise WeChat Markdown editor. |
|
7 | technical-books | A list of technical books worth reading. |
|
Contributor
Thanks to all the following friends for their contributions to the Doocs technical community. Please click here to participate in project maintenance.
Official account
The only public account " Doocs " under the Doocs technology community, welcome to scan the QR code to follow, focusing on sharing relevant knowledge in the technical field and the latest industry information . Of course, you can also add my personal WeChat account (note: GitHub) to bring you into the technical exchange group.
Follow the " Doocs " public account and reply to the PDF to get the offline PDF document of this project (283 pages of essence), making learning more convenient!
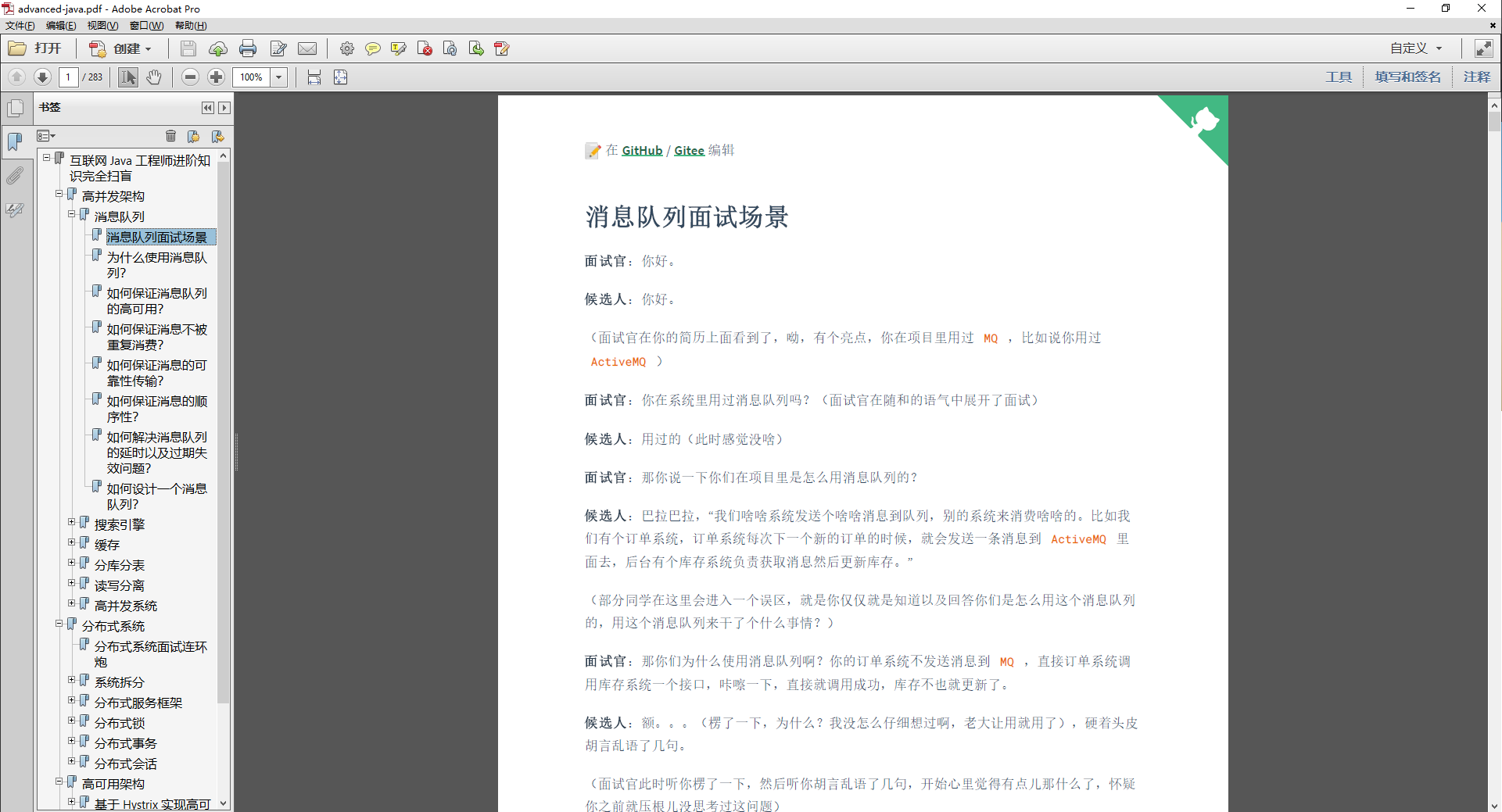