This article brings you relevant knowledge about JavaScript. It mainly introduces related issues about BOM operations, including common events of window objects, JavaScript execution mechanisms, etc. Let’s take a look at it together. I hope it will be helpful to everyone. Helpful.
Common events of the window object
Window loading event:
- window.onload: page loading event. This event is triggered when the document content is completely loaded (including images, script files, CSS files, etc.) and the processing function is called.
- document.addEventListener('DOMContentLoaded', function(){}): Only when the DOM loading is completed, excluding style sheets, pictures, flash, and compatibility
events to adjust the window size:
- window.onresize: Adjust the window size loading event
window The .open() method can be used to navigate to a specified URL or to open a new browser window.
- This method receives 4 parameters: the URL to be loaded, the target window, a character string and a representation of the new window in the browser history. Whether to represent the Boolean value of the currently loaded page
window.open("http://www.wrox.com/", "wroxWindow","height=400,width=400,top=10,left=10,resizable=yes");
timer:
- setInterval(handler: any, timeout?: long, arguments…: any): loop
- clearInterval(handle?: long): cancel setInterval
- setTimeout(handler: any, timeout?: long, arguments…: any) : One-time
- clearTimeout(handle?: long): Cancel setTimeout
window.scroll(x, y)
window.scrollTo(x, y): Both are the same usage to change the position of the horizontal and vertical scroll bars, provided that there must be The scroll bar is on the page
window.scrollBy(x, y): The cumulative scrolling of the scroll bar, positive numbers go down, negative numbers go up window.scrollBy(0, 10): When called every 100 milliseconds, the scroll bar moves 10 pixels
window.getComputedStyle(elem, pseudo-class)
dialog box
- alert
- alert("prompt string")
- pops up a warning box and displays the prompt string text in the warning box
- confirm
- confirm("prompt string")
- displays a confirmation box and confirms The prompt string is displayed in the box.
- When the user clicks the "Confirm" button, it returns true, and clicks "Cancel" to return false
- prompt
- prompt ("prompt string", "default value")
- displays an input box and displays the prompt character in the input box String, waiting for user input.
- When the user clicks the "Confirm" button, the user input is returned. When the "Cancel" button is clicked, a null value is returned.
The JavaScript execution mechanism
runs the js script and puts the js code into the execution stack in a synchronous execution mode. When running the execution stack, it encounters JS asynchronous code (events, timers, ajax, resource loading, error) is put into web APIs (task queue). When the code in the execution stack is completed, go to the task queue and take the first one for execution. After execution, Get one from the task queue and execute it, and execute it repeatedly (event loop) until the execution in the task queue is completed.
The location object
window.history is used to obtain the address URL of the current page and redirect the browser to a new page.
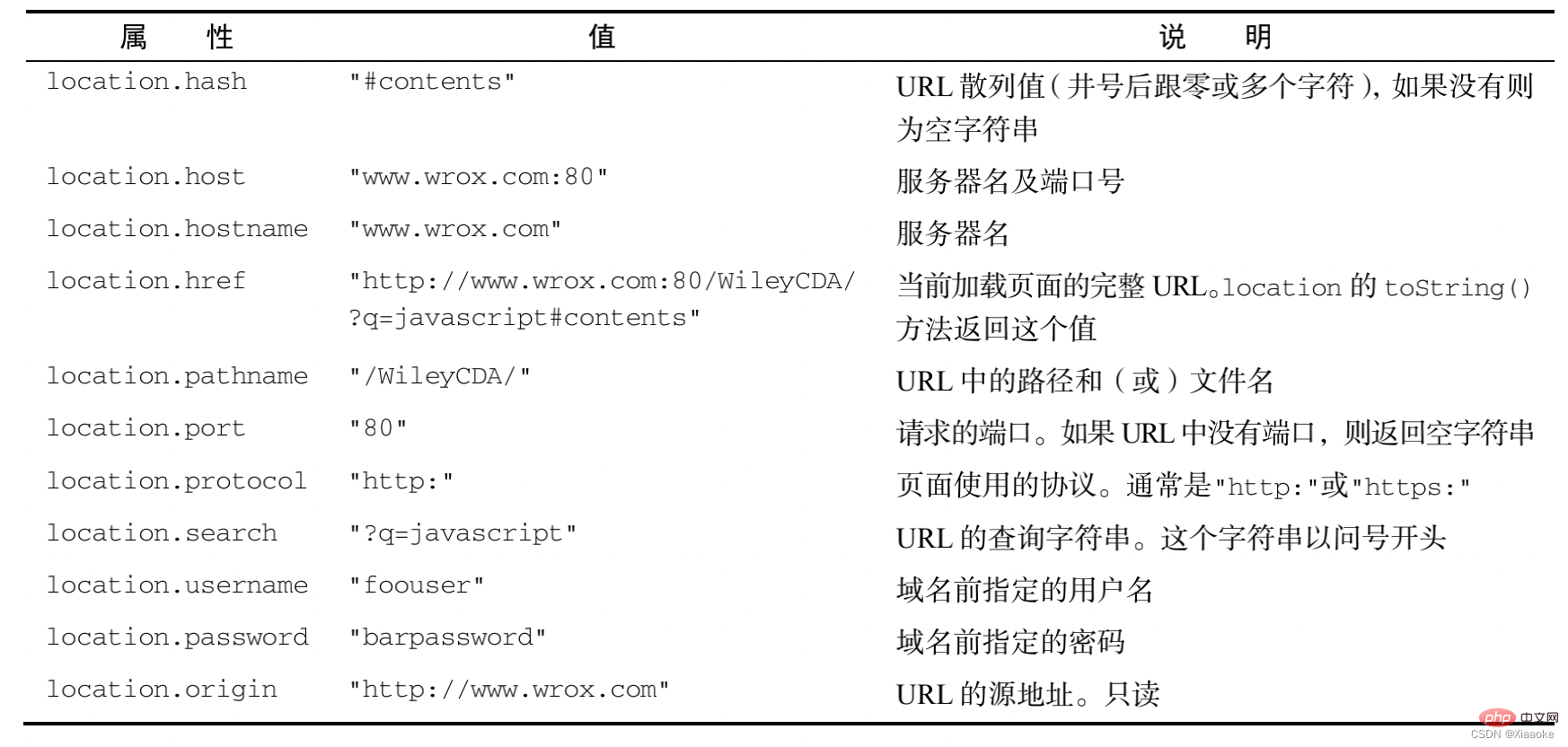
http://www.itcast.cn:80/index.html?name=andy&age=1#link
http: communication protocol www.itcast.cn: domain name 80: port index.html: path?name=andy&age=1: parameter #link fragment: anchor point, link
object attribute:
- href*: get or set the entire URL
- host: return the host Name (domain name)
- hostname: Set or return the host name of the current URL
- post: Return the port number
- pathname: Return the path
- search*: Return parameter
- hash: Return the fragment (content after #)
- protocol: Set or return the protocol
object method of the current URL:
- assign: Like href, you can jump to the page (also called a redirect page)
- replace: replace the current page, because the history is not recorded, you cannot go back to the page
- reload: reload the page, equivalent to the refresh function
navigator object
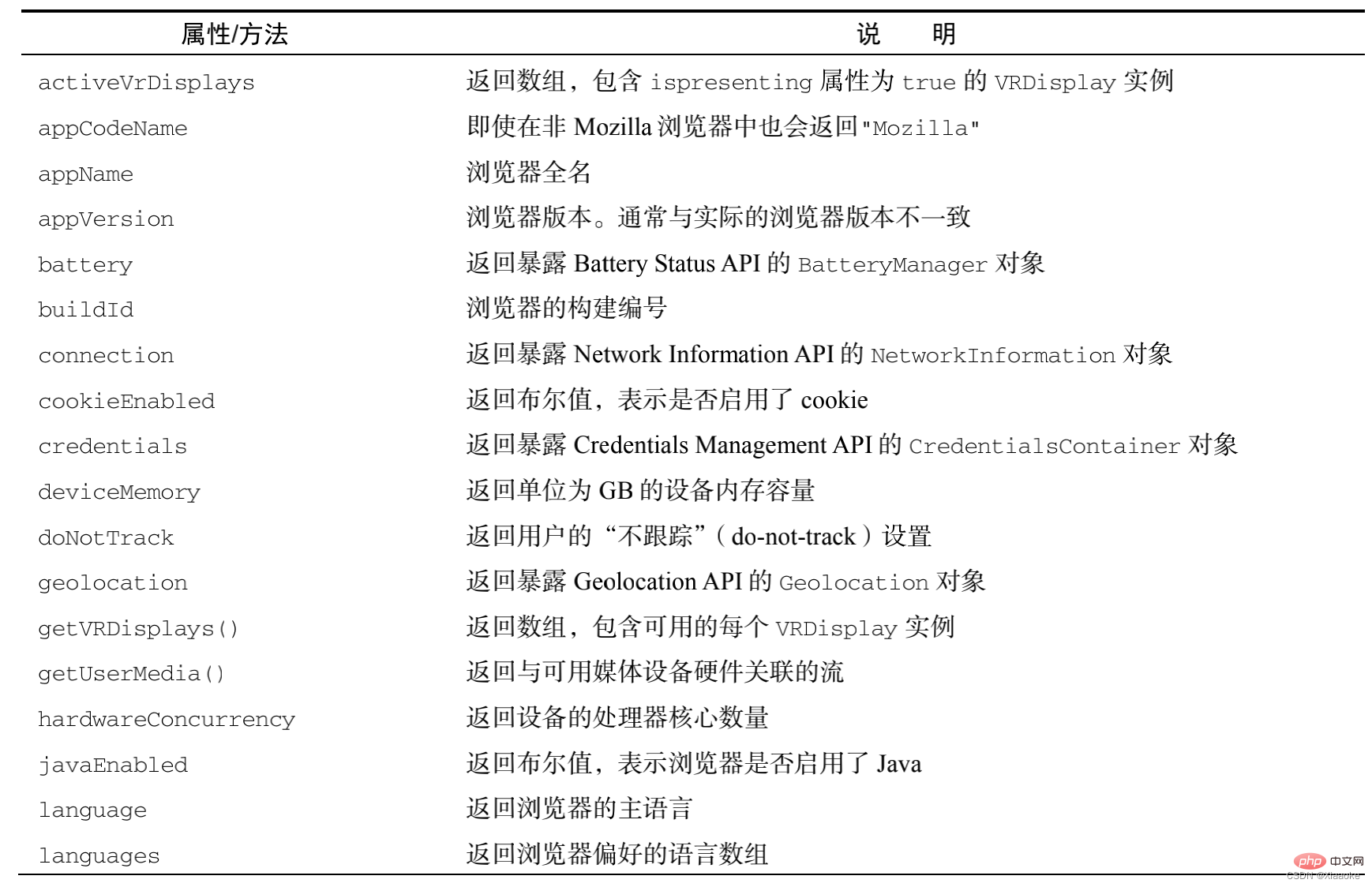
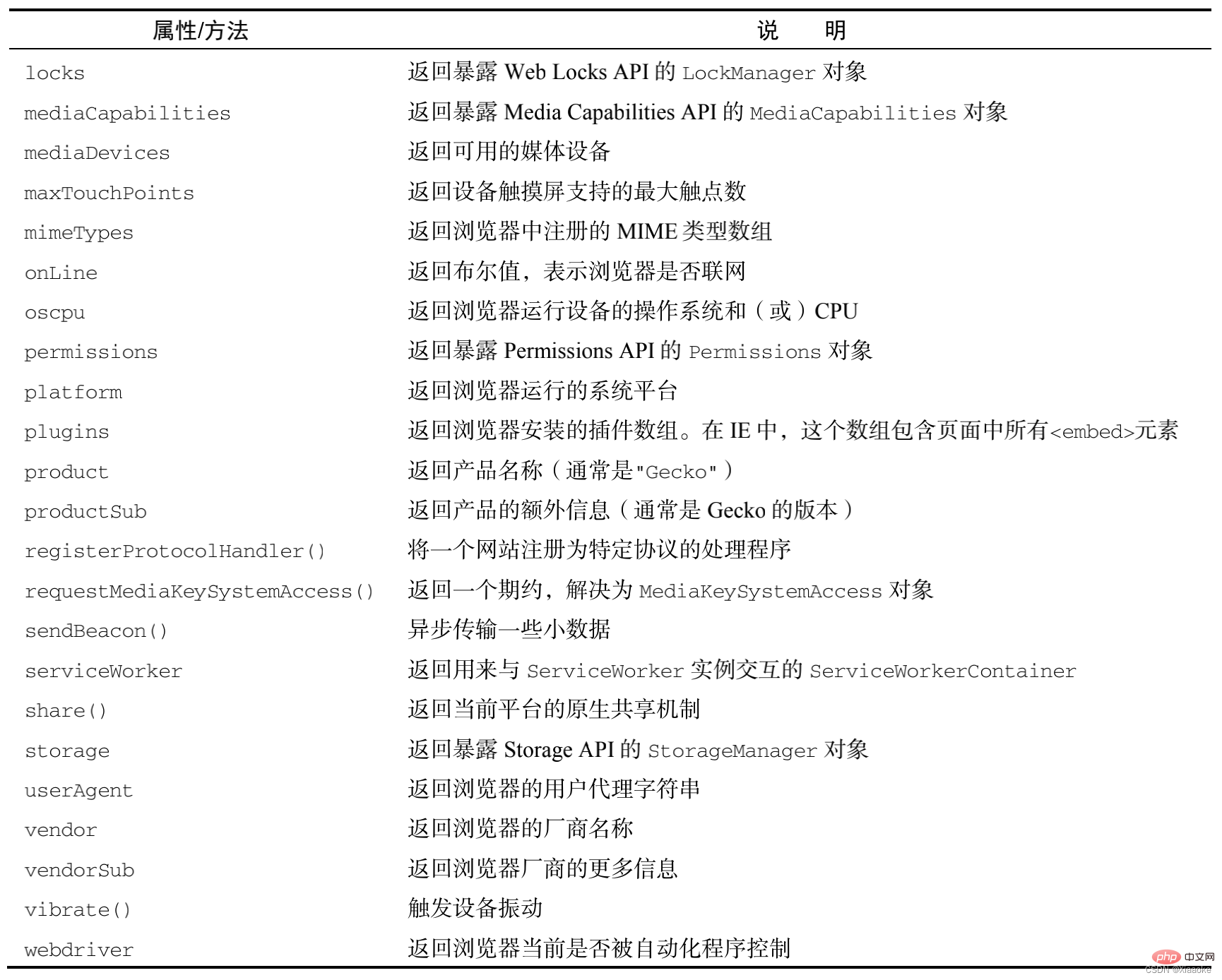
navigator: an object that encapsulates browser configuration information
- cookieEnabled Whether cookies are turned on in the current browser
- cookie: The storage space on the client, and the capacity is small. Different browsers have different sizes, and the key can be saved permanently
- Disadvantages: Particularly easy Leak personal information
- plugins encapsulate all plug-in information installed by the browser
- userAgent browser name, kernel version number and other series of characters
- onLine Is the computer offline? Is the computer connected to the Internet?
- platform returns the operating system platform running the browser
- appCodeName returns the code name of the browser
- appName returns the name of the browser
- appVersion returns the platform and version information of
the browser history object
window.history object includes the browser's history (url) collection
- browser's back Function: history.back()
- Browser's forward function: history.forward()
- Enter a certain page in the history: history.go()
screen object
window.screen object contains information about the user
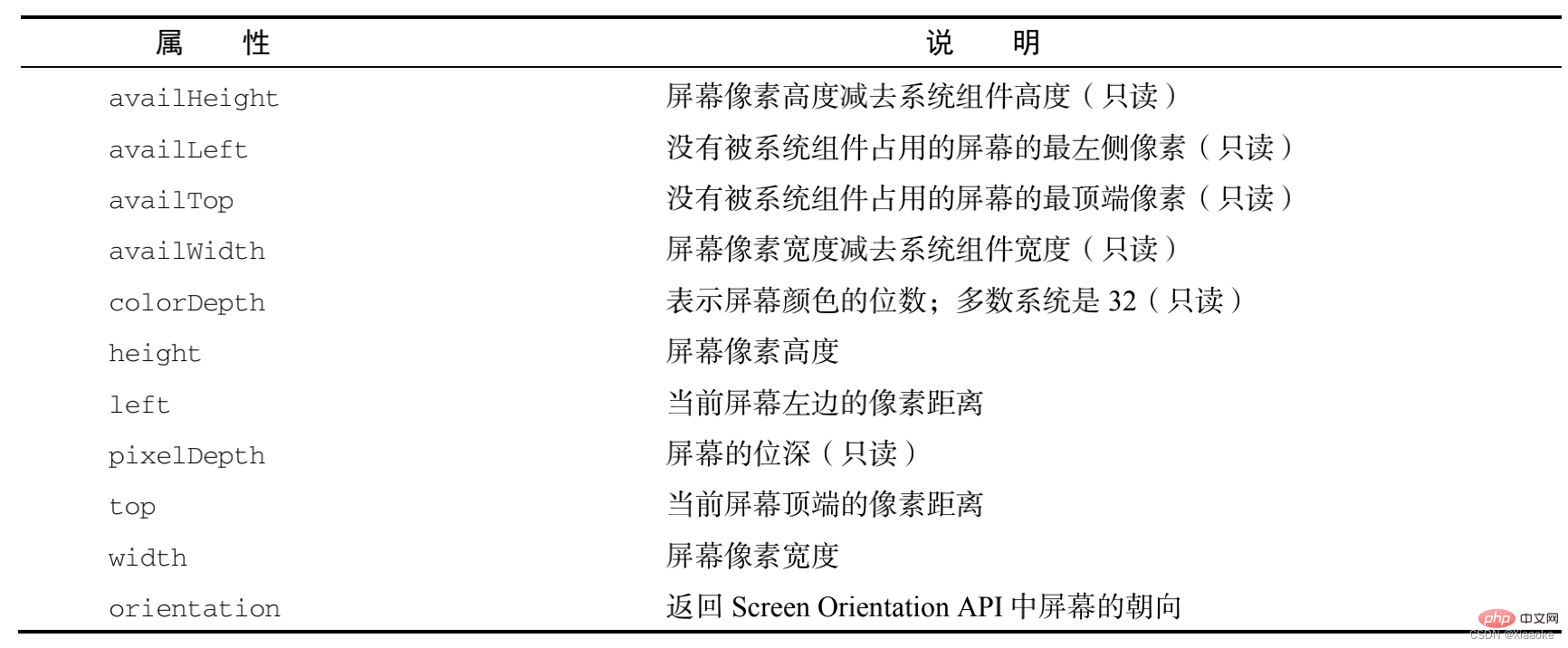
// screen: Get the resolution size of the display device // Full resolution: screen.widht/height
// How to identify the type of client compatible with all client widths // Large screen, medium screen, small screen, ultra-small screen // lg md sm xs
// TV pc pad phone
//Width >= 1200 >=992 >= 768 < 768
//The remaining resolution after removing the taskbar // screen.availHeight/availWidth
- Available screen width: screen.availWidth
- Available screen height: screen.availHeight
- Screen height: screen.Height
- Screen width: screen.Width
- The number of digits of the screen color : colorDepth
element offset The offset series
can dynamically obtain the position (offset), size, etc. of the element.
- Get the distance of the element to the position of the parent element.
- Get the size of the element itself
. - ps: The returned value does not have a unit.
Commonly used attributes of the offset series:
- element. offsetParent: Returns the parent element with positioning as the element. If the parent element is not positioned, the body is returned.
- element.offsetTop*: Returns the offset of the element above the positioned parent element.
- element.offsetLeft*: Returns the relative band of the element. There is an offset to the left of the positioned parent element
- element.offsetWidth: Returns the width of itself including padding, border, and content, without unit
- element.offsetHeight: Returns the height of itself including padding, border, and content, without unit
element visual area client The series
dynamically obtains common attributes
such as the border size and element size of elements
: - element.clientTop: the size of the upper border of the element
- element.clientLeft: the size of the left border of the element
- element.clientWidth*: returns the width of itself including padding and content area, excluding the border , without unit
- element.clientHeight*: Returns the height of itself including padding and content area, excluding borders, without unit
element scroll scroll series
dynamically obtains the size and scroll distance of elements.
Common attributes
- element.srcollTop*: returns the rolled Upper side distance, without unit
- element.srcollLeft*: Returns the rolled left distance, without unit
- element.srcollWidth: Returns its actual width, without border, without unit
- element.srcollHeight: Returns its actual height , does not contain borders and does not have a unit
scroll bar. When scrolling, the onscroll event will be triggered
to view the scrolling distance of the scroll bar.
window.pageXOffset/pageYOffset
IE8 and below are not compatible with document.body/documentElement.scrollLeft/scrollTop
The compatibility is confusing and takes a long time. Add two values, because it is impossible for two values to have values at the same time . Encapsulation compatibility method, find the rolling distance of the scroll bar wheel getScrollOffet()
/*
Encapsulates a method to get the scrolling distance of the scroll bar. Returns: x: the scrolling distance of the horizontal scroll bar y: the scrolling distance of the vertical scroll bar */function getScrollOffet(){
if(window.pageXOffset){
return {//{} of the object must be after the keyword, otherwise the system will automatically add it; then the return value will be undefined
x : window.pageXOffset,
y : window.pageYOffset }
}else{//Compatible with IE8 and below return {
x : document.body.scrollLeft + document.documentElement.scrollLeft,
y : document.body.scrollTop + document.documentElement.scrollTop }
}}
View the size of the viewport
window.innerWidth/innerHeight
is not compatible with IE8 and below (note: the width and height here do not include the height of the menu bar, toolbar, scroll bar, etc.) document.documentElement.clientWidth/clientHeight
in standard mode , any browser is compatible with document.body.clientWidth/clientHeight
It is a browser encapsulation compatibility method suitable for weird situations. Returns the browser viewport size getViewportOffset()
/*Encapsulation returns the browser viewport size return value:
w: width of the viewport h: height of the viewport*/function getViewportOffset(){
if(window.innerWidth){
return {
w : window.innerWidth,
h : window.innerHeight }
}else{ //Compatible with IE8 and below browsers if(document.compatMode == 'BackCompat'){
//Return { in weird rendering mode
w : document.body.clientWidth,
h : document.body.clientHeight }
}else{
//Standard mode return {
w : document.documentElement.clientWidth,
h : document.documentElement.clientHeight }
}
}}console.log(document.compatMode); // BackCompat weird mode // CSS1Compat standard mode
to view the geometric size of the element. ES5 new understanding of
domElement.getBoundingClientRect()
has good compatibility; returns an object, which contains left, Attributes such as top, right, and bottom. Left and top represent the X and Y coordinates of the upper left corner of the element. Right and bottom represent the X and Y coordinates of the lower right corner of the element. The height and width attributes are not implemented in old versions of IE. The returned results are not real-time. '
// Get the position of the element in the document function getElementPosition(target){
//Support BoundingClientRect() method if(0 && target.getBoundingClientRect){
var pos = target.getBoundingClientRect();
return { // When the scroll bar moves, add the position x of the scroll bar: pos.left + Math.max(document.body.scrollLeft, document.documentElement.scrollLeft),
y : pos.top + Math.max(document.body.scrollTop, document.documentElement.scrollTop)
}
} else {
var pos = {
left: 0,
top : 0
}
var _elm = target;
while(target.offsetParent){
if(_elm == target){//Accumulate left and top for the first time
pos.left += target.offsetLeft;
pos.top += target.offsetTop;
}else{
pos.left += target.offsetLeft + target.clientLeft;
pos.top += target.offsetTop + target.clientTop;
}
// target reassigns target = target.offsetParent;
}
return { x : pos.left, y : pos.top}
}}
Property
status bar
- defaultStatus changes the default display status of the browser status bar
- temporarily changes the display
window position
of the browser status - IE
- screenLeft declares the x coordinate of the upper left corner of the window
- screenTop declares the y coordinate of the upper left corner of the window
- document.body.screenLeft
- document. documentElement.screenLeft declares the number of pixels the current document has scrolled to the right
- document.body.screenTop
- document.documentElement.screenTop declares the number of pixels the current document has scrolled to the right
- ! IE
- screenX declares the x-coordinate of the upper left corner of the window
- screenY declares the upper left corner of the window The y coordinate
- pageXOffset declares the number of pixels that the current document has scrolled to the right
- pageYOffset declares the number of pixels that the current document has scrolled to the right
- FF
- innerHeight returns the height of the document display area of the
- window innerWidth returns the width of the document display area of the window
- outerWidth returns the outer width of the window
- outerHeight Returns the outer height of the window
and other attributes
- opener can realize communication between cross-forms under the same domain name. A form should contain the opener of another form.
- closed returns true when the current window is closed.
- name sets or returns the name of the window.
- self returns the name of the current window. Quote