Algorithm Clearance Manual (LeetCode)
01. Project Introduction
A tutorial to explain the basic knowledge of "Algorithm and Data Structure" , and a detailed analysis of 800+ questions in "LeetCode". This project is easy to understand and there are no big leaps in thinking. Some illustrations and examples are used in the project to help understanding.
This tutorial starts with basic data structures and algorithms, and then explains and analyzes specific topics for different categories of data structures and algorithms. It allows readers to thoroughly master algorithm knowledge through a combination of "basic theoretical learning of algorithms" and "practical programming learning".
This tutorial uses Python as the programming language and requires learners to have basic knowledge and experience in Python programming.
02. Project address
Welcome "Star ️" and "Fork" in the upper right corner, this is my greatest encouragement and support.
- GitHub address: https://github.com/itcharge/LeetCode-Py
An online e-book "Algorithm Clearance Manual" that supports dark mode.
- E-book address: https://algo.itcharge.cn
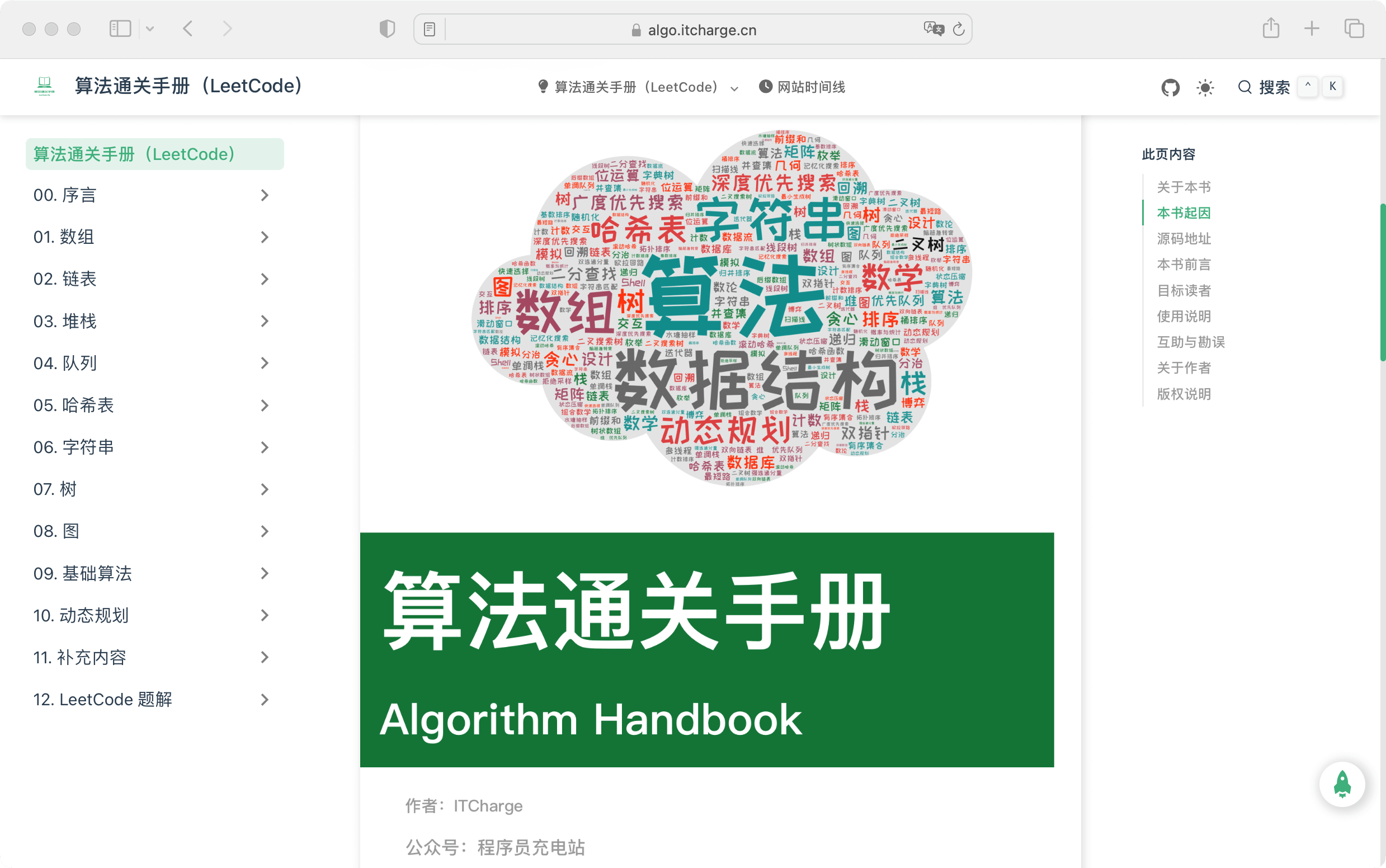
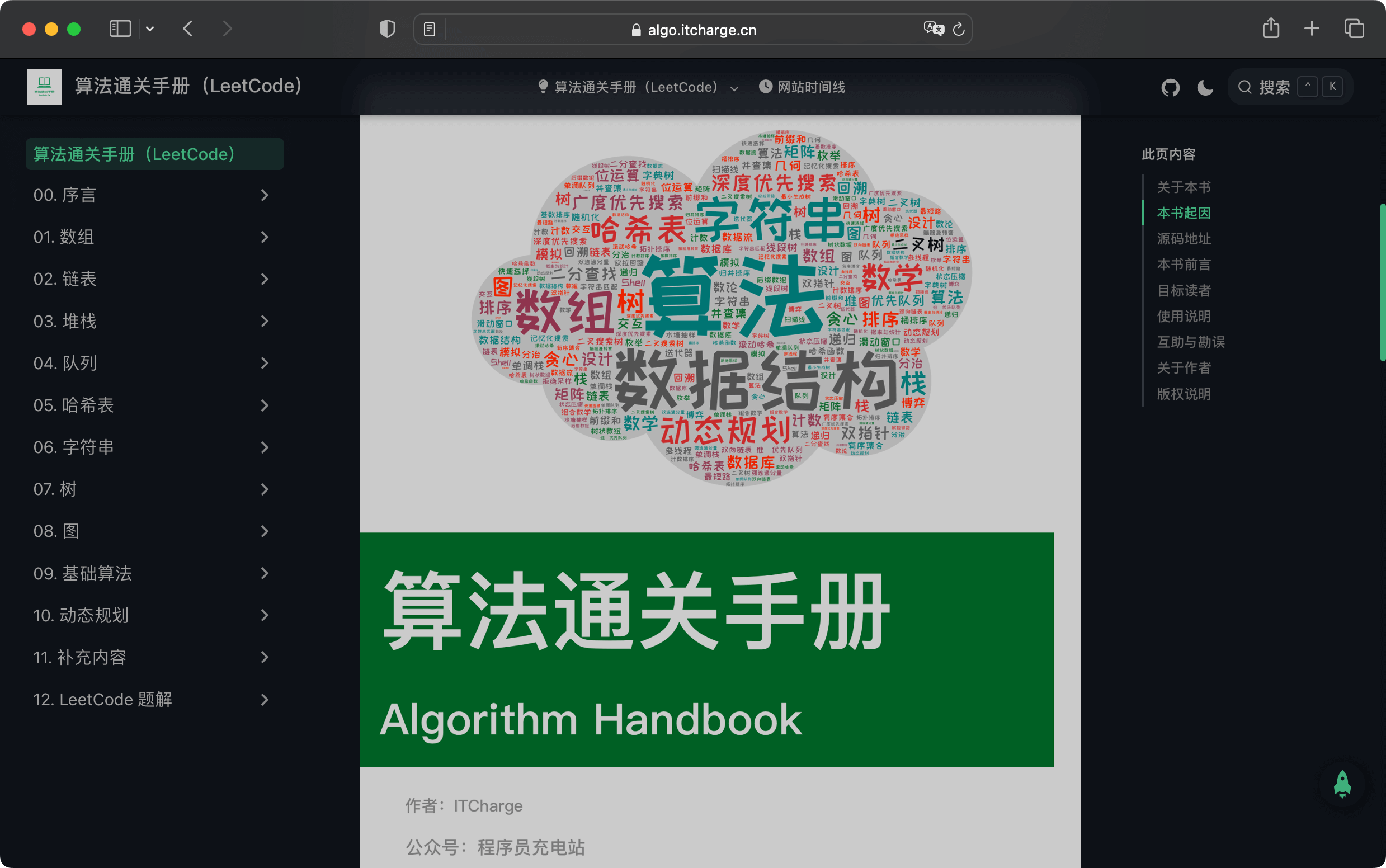
03. About the author
I am an iOS/macOS development programmer, and I am also a part-time master's student (currently studying) at Beihang Software Institute. I studied algorithm knowledge in college and participated in ACM competitions for three years, but my level was limited and I failed to achieve ideal results. But the biggest gain for me from these three years of ACM experience is that I have developed my logical thinking and ability to solve practical problems. This ability has laid a solid foundation for my future work and study.
I started solving questions on LeetCode every day on March 30, 2021. By June 8, 2022, I had solved 1,000+ questions and completed 800+ problem solutions. Work hard to solve 1000+, 1500+, 2000+ questions.
Reply " Algorithm Check-in " in the public account "Programmer Charging Station" , and you will be added to the LeetCode algorithm check-in plan group to form a team and check in together.
- Password to enter the group: Algorithm check-in
- Requirements for joining the group: less chatting, more sharing, and changing notes.
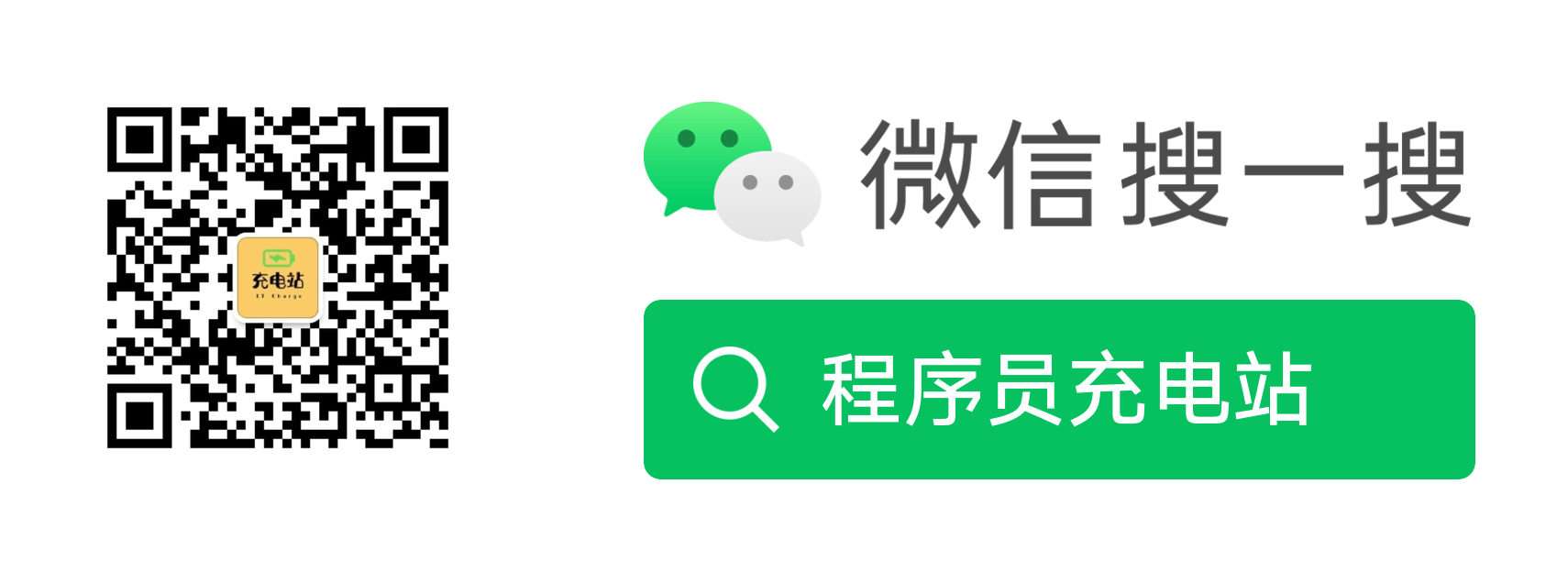
04. Copyright statement
- This tutorial is licensed under a Creative Attribution-NonCommercial-NoDerivs (BY-NC-ND) 4.0 International License.
- The copyright of all the questions in this tutorial belongs to LeetCode and LeetCode China.
05. Chapter Table of Contents
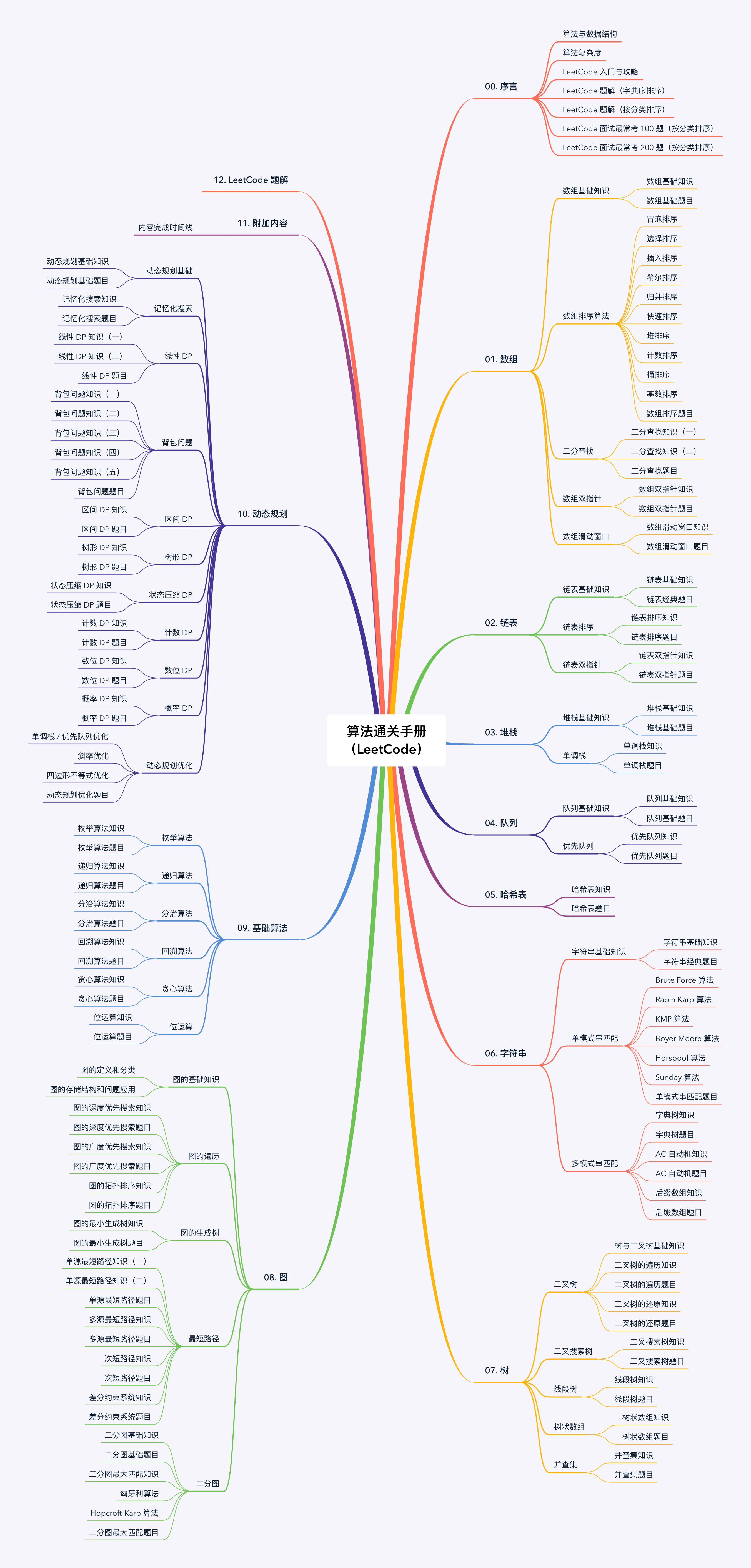
00. Introduction
- Algorithms and Data Structures
- Algorithmic complexity
- Getting started with LeetCode and strategies
- LeetCode problem solutions (lexicographic sorting, 850+ problem solutions)
- LeetCode problem solutions (sorted by category, recommended problem list ★★★)
- LeetCode's 100 most frequently asked interview questions (sorted by category)
- The 200 most frequently asked questions in LeetCode interviews (sorted by category)
01. Array
- Array basics
- Array basics
- Array basic questions
- Array sorting algorithm
- bubble sort
- selection sort
- insertion sort
- Hill sort
- merge sort
- Quick sort
- Heap sort
- counting sort
- bucket sort
- Radix sort
- Array sorting question
- binary search
- Binary search knowledge (1)
- Binary search knowledge (2)
- Binary search question
- Array double pointer
- Array double pointer knowledge
- Array double pointer question
- Array sliding window
- Array sliding window knowledge
- Array sliding window question
02. Linked list
- Basic knowledge of linked lists
- Basic knowledge of linked lists
- Classic linked list questions
- Linked list sorting
- Linked list sorting knowledge
- Linked list sorting question
- Linked list double pointer
- Knowledge about linked list double pointers
- Linked list double pointer question
03. Stack
- Stack basics
- Stack basics
- Stack basic questions
- monotonic stack
- Monotone stack knowledge
- Monotone stack question
04. Queue
- Queue basics
- Queue basics
- Queue basic questions
- priority queue
- Priority queue knowledge
- priority queue question
05. Hash table
- Hash table knowledge
- Hash table question
06. String
- String basics
- String basics
- String classic questions
- Single pattern string matching
- Brute Force Algorithm
- Rabin Karp algorithm
- KMP algorithm
- Boyer Moore algorithm
- Horspool algorithm
- Sunday Algorithm
- Single pattern string matching question
- Multiple pattern string matching
- Dictionary tree knowledge
- Dictionary tree question
- AC automatic machine knowledge
- AC automaton problem
- Suffix array knowledge
- Suffix array question
07. Tree
- Binary tree
- Basic knowledge of trees and binary trees
- Binary tree traversal knowledge
- Binary tree traversal problem
- Binary tree restoration knowledge
- Binary tree restoration problem
- binary search tree
- Binary search tree knowledge
- Binary search tree question
- Segment tree
- Segment tree knowledge
- Line segment tree question
- tree array
- Tree array knowledge
- Tree Array Question
- And search the collection
- and search for knowledge
- And check the questions
08. Graph theory
- Basic knowledge of graphs
- Definition and classification of graphs
- Graph storage structure and problem application
- Graph traversal
- Depth-first search for knowledge on graphs
- Depth first search problem for graphs
- Breadth-first search for knowledge in graphs
- Graph breadth first search question
- Topological sorting knowledge of graphs
- Graph topological sorting question
- Spanning tree of graph
- Minimum spanning tree knowledge for graphs
- Minimum spanning tree problem for graphs
- shortest path
- Single source shortest path knowledge (1)
- Single source shortest path knowledge (2)
- Single source shortest path problem
- Multi-source shortest path knowledge
- Multi-source shortest path problem
- Shortest path knowledge
- Second short path question
- Differential constraint system knowledge
- Differential constraint system questions
- bipartite graph
- Basic knowledge of bipartite graphs
- Basic questions on bipartite graphs
- Bipartite graph maximum matching knowledge
- Hungarian algorithm
- Hopcroft-Karp algorithm
- Bipartite graph maximum matching problem
09. Basic algorithm
- Enumeration algorithm
- Enumeration algorithm knowledge
- Enumeration algorithm questions
- recursive algorithm
- Recursive algorithm knowledge
- Recursive Algorithm Questions
- divide and conquer algorithm
- Divide and conquer algorithm knowledge
- Divide and Conquer Algorithm Questions
- Backtracking algorithm
- Backtracking algorithm knowledge
- Backtracking algorithm questions
- greedy algorithm
- Greedy algorithm knowledge
- Greedy Algorithm Question
- Bit operations
- Bit operation knowledge
- Bit operation questions
10. Dynamic programming
- Dynamic programming basics
- Dynamic programming basics
- Basic questions on dynamic programming
- Memoized search
- Memory search knowledge
- Memory search questions
- Linear DP
- Linear DP knowledge (1)
- Linear DP knowledge (2)
- Linear DP question
- backpack problem
- Knapsack problem knowledge (1)
- Knowledge about backpack problems (2)
- Knowledge about backpack problems (3)
- Knowledge about Backpack Problem (4)
- Knowledge about backpack problems (5)
- knapsack problem questions
- Interval DP
- Interval DP knowledge
- Interval DP question
- Tree DP
- Tree DP knowledge
- Tree DP question
- State Compression DP
- State compression DP knowledge
- State Compression DP Question
- Count DP
- Counting DP Knowledge
- Counting DP questions
- Digital DP
- Digital DP knowledge
- Digital DP questions
- Probability DP
- Probability DP knowledge
- Probability DP questions
- Dynamic programming optimization
- Monotone stack/priority queue optimization
- Slope Optimization
- Quadrilateral inequality optimization
- Dynamic programming optimization problem
11. Additional content
- Content completion timeline
12. LeetCode problem solutions (860 questions completed)