Recommended to read online (Github access is often unstable in the country)
Recommended Gitee synchronization
- Introduction : This project is a complete set of test plan, designed to help everyone avoid detours, learn algorithms step by step, and follow the author.
- Officially published : "Code Thoughts".
- PDF version : PDF version of "Code Random Notes" on algorithms.
- Algorithm open class : "Code Random Record" algorithm video open class.
- The strongest eight-part essay : Code Random Notes on the Essence of the Knowledge Planet PDF.
- The order of answering questions : The README has arranged the order of answering questions. You can just answer them one by one in order.
- Learning community : Learn together about check-in/interview skills/how to choose an offer/recommendations from large companies/workplace rules/resume modification/technology sharing/program life. Welcome to join the "Code Caprice" knowledge planet.
- Submit code : This project uses C++ language to explain, but there are already multi-language versions such as Java, Python, Go, JavaScript, etc. Thank you to every contributor here. If you also want to contribute code to light up your avatar, click Learn how to submit your code here.
- Note for reprinting : All the following articles are original works by me (programmer Carl). Please indicate the source when quoting articles from this project. If you find malicious plagiarism or transfer, you will use legal weapons to protect your rights and interests. Let us maintain a good technical creation environment together!
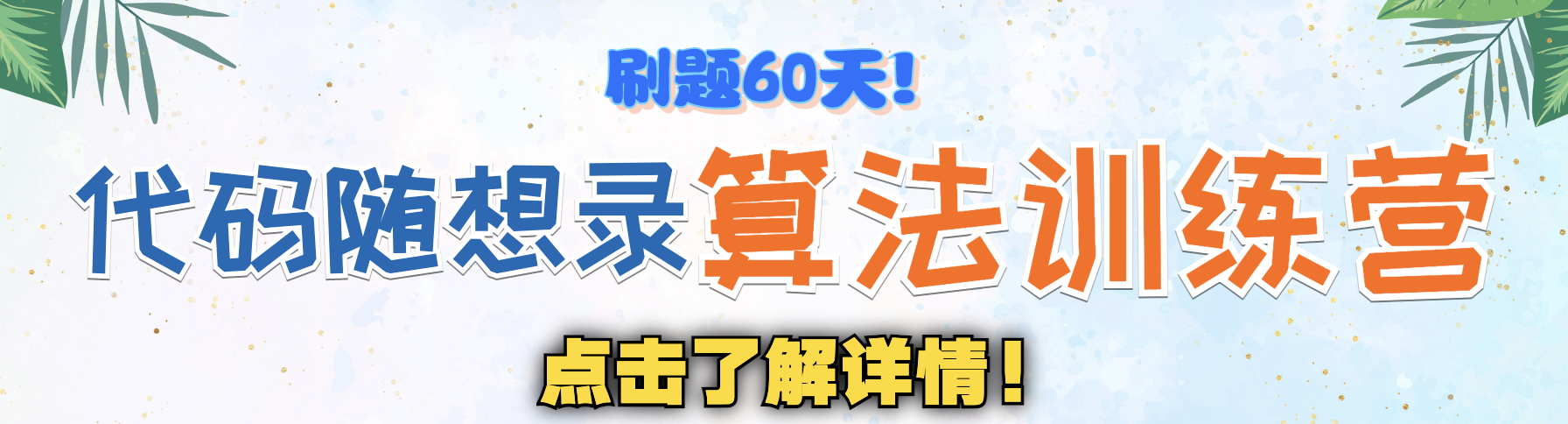
LeetCode question brushing guide
Background of the question brushing guide
Many students who have just started to answer questions have a confusion: facing nearly 2,000 questions on leetcode, where to start.
Everyone feels that the efficiency of answering questions is inefficient, and the time wasted mainly on three points:
- Find questions
- Found a question that should not be done at this stage
- There is no complete set of high-quality problem solutions for reference.
In fact, I have answered this question before on Zhihu. The answer is roughly as follows: array-> linked list-> hash table-> string-> stack and queue-> tree-> backtracking-> greedy-> Dynamic Programming -> Graph Theory -> Advanced Data Structures, then start with the simple ones, and after doing a few types of questions, gradually move on to medium and difficult questions.
But I can put myself in my shoes and feel: Even with such an overall plan, it is very difficult for a beginner or even an algorithm veteran to find a topic that suits him. The time cost is very high, and the topic is not necessarily a classic topic.
When it comes to answering questions, we all want to use the shortest time to do all the classic questions in a step-by-step order of difficulty , so that the efficiency is the highest!
So I compiled a guide for clearing Leetcode questions: a super detailed order of answering questions. Each question is carefully selected by me. They are all classic and high-frequency interview questions . You just need to follow this order. You read that right. The README has arranged the order of the questions, and the order of the articles is the order of answering the questions! Just brush them one by one, you don’t have to go through the sea of questions and choose the topics yourself!
Moreover, I have written detailed solutions to each question (with pictures and texts, and videos on difficult points). My solutions are listed on the homepage of the corresponding questions, and the quality is obvious to all.
So now I have sorted out the order of answering questions to help more students who are learning algorithms avoid detours!
If you are brushing leetcode, it is strongly recommended to follow the order of solving the questions in this guide. After finishing the brushing, you will find that you have made a qualitative leap in the entire knowledge system, and you don’t have to look for directions in the sea of questions.
The latest articles will be published first on the public account "Code Caprice". Scan the code to take a look, and you will find that it is too late to meet you!
How to use this quiz guide
According to the order of arrangement mentioned above, just start brushing from the array. The order has been arranged, so just brush in order.
In the question brushing guide, each topic has a theoretical foundation chapter at the beginning. It is not like a textbook-like theoretical introduction, but a summary of the basic knowledge needed from actual combat. There is a summary at the end of each topic, which is the most comprehensive summary of the topic.
If you are a veteran of algorithms, this guide is also the best material for review. If you quickly read through the summary chapter corresponding to each series, the entire algorithm knowledge system and various solutions will come back to your mind.
Every solution here is a masterpiece and deserves careful consideration .
I use C++ uniformly in the problem explanations, but you will find that almost every problem explanation below is equipped with other language versions, such as Java, Python, Go, JavaScript, etc. It is the code contributed by these enthusiastic guys. Of course, I will also strictly Control code quality.
Therefore, everyone is welcome to participate, improve the various language versions of the problem solutions, embrace open source, and benefit more friends .
Are you ready? Let’s start the quiz guide, go go go!
Preface
array
- Arrays are too simple, but you should know this!
- Array: 704.Binary search
- Array: 27. Remove elements
- Array: 977. Square of ordered array
- Array: 209. Subarray with minimum length
- Array: range sum
- Array: Developer purchases land
- Array: 59. Spiral Matrix II
- Arrays: Summary
linked list
- Here’s what you should know about linked lists!
- Linked list: 203. Remove linked list elements
- Linked list: 707. Design linked list
- Linked list: 206. Flip the linked list
- Linked list: 24. Exchange nodes in the linked list pairwise
- Linked list: 19. Delete the Nth node from the bottom of the linked list
- Linked list: Linked list intersects
- Linked list: 142. Circular linked list
- Linked List: Summary!
Hash table
- What you should know about hash tables!
- Hash table: 242. Valid anagrams
- Hash table: 1002. Find common characters
- Hash table: 349. Intersection of two arrays
- Hash table: 202.Happy number
- Hash table: 1. Sum of two numbers
- Hash table: 454. Addition of four numbers II
- Hash table: 383. Ransom letter
- Hash table: 15. Sum of three numbers
- Two-pointer method: 18. Sum of four numbers
- Hash table: summary!
string
- String: 344. Reverse string
- String: 541. Reverse String II
- String: replace numbers
- String: 151. Flip the words in the string
- String: right-handed string
- Help you learn the KMP algorithm thoroughly
- String: 459. Repeated substring
- String: Summary!
double pointer method
The double pointer method is basically applied to problems with arrays, strings and linked lists.
- Array: 27. Remove elements
- String: 344. Reverse string
- String: replace numbers
- String: 151. Flip the words in the string
- Linked list: 206. Flip the linked list
- Linked list: 19. Delete the Nth node from the bottom of the linked list
- Linked list: Linked list intersects
- Linked list: 142. Circular linked list
- Double pointers: 15. Sum of three numbers
- Double pointers: 18. Sum of four numbers
- Double Pointers: Summary!
Stacks and Queues
- Stacks and Queues: Theoretical Basics
- Stack and queue: 232. Use stack to implement queue
- Stack and queue: 225. Use queue to implement stack
- Stacks and Queues: 20. Valid Parentheses
- Stacks and Queues: 1047. Remove all adjacent duplicates in a string
- Stacks and Queues: 150. Reverse Polish Expression Evaluation
- Stacks and queues: 239. Maximum sliding window
- Stack and queue: 347. Top K high-frequency elements
- Stacks and Queues: Summary!
Binary tree
The topic classification outline is as follows:
- Here’s what you should know about binary trees!
- Binary Tree: Recursive Traversal of Binary Tree
- Binary tree: iterative traversal of a binary tree
- Binary Tree: Unified Iteration Method for Binary Trees
- Binary tree: level-order traversal of a binary tree
- Binary Tree: 226. Flip Binary Tree
- Summary of this week! (binary tree)
- Binary Tree: 101. Symmetric Binary Tree
- Binary tree: 104. The maximum depth of a binary tree
- Binary tree: 111. Minimum depth of binary tree
- Binary tree: 222. The number of nodes in a complete binary tree
- Binary Tree: 110. Balanced Binary Tree
- Binary tree: 257. All paths of the binary tree
- Wrapping up this week! (binary tree)
- Binary tree: 404. Sum of left leaves
- Binary tree: 513. Find the value in the lower left corner of the tree
- Binary tree: 112. Path sum
- Binary tree: 106. Construct a binary tree
- Binary tree: 654. Maximum binary tree
- Summary of this week! (binary tree)
- Binary Tree: 617. Merge two binary trees
- Binary tree: 700. Binary search tree appears!
- Binary Tree: 98. Verify Binary Search Tree
- Binary tree: 530. Minimum absolute difference of search tree
- Binary Tree: 501. Mode in Binary Search Tree
- Binary Tree: 236. Common Ancestor Problem
- Summary of this week! (binary tree)
- Binary Tree: 235. Search for the nearest common ancestor of the tree
- Binary Tree: 701. Insertion Operation in Search Tree
- Binary tree: 450. Delete operation in search tree
- Binary Tree: 669. Pruning Binary Search Tree
- Binary Tree: 108. Convert ordered array to binary search tree
- Binary tree: 538. Convert binary search tree to cumulative tree
- Binary Tree: Summary! (All the binary tree skills you need to master are here)
Backtracking algorithm
The topic classification outline is as follows:
- Here’s what you should know about backtracking algorithms!
- Backtracking Algorithm: 77. Combination
- Backtracking Algorithm: 77. Combinatorial Optimization
- Backtracking Algorithm: 216. Combinatorial Sum III
- Backtracking Algorithm: 17. Alphabet combination of phone numbers
- Summary of this week! (Backtracking algorithm series one)
- Backtracking Algorithm: 39. Combinatorial Sum
- Backtracking Algorithm: 40. Combinatorial Sum II
- Backtracking algorithm: 131. Split palindrome string
- Backtracking algorithm: 93. Restore IP address
- Backtracking Algorithm: 78.Subset
- Summary of this week! (Backtracking algorithm series 2)
- Backtracking Algorithm: 90. Subset II
- Backtracking algorithm: 491. Increasing subsequence
- Backtracking algorithm: 46. Full permutation
- Backtracking Algorithm: 47. Total Permutation II
- Summary of this week! (Backtracking algorithm series three)
- Another way to write the backtracking algorithm to remove duplicates
- Backtracking algorithm: 332. Rearrange itinerary
- Backtracking algorithm: 51.N Queen
- Backtracking Algorithm: 37. Solve Sudoku
- Summary of Backtracking Algorithm
greedy algorithm
The topic classification outline is as follows:
- What you should know about greedy algorithms!
- Greedy algorithm: 455. Distribute cookies
- Greedy algorithm: 376. Swing sequence
- Greedy algorithm: 53. Maximum subsequence sum
- Summary of this week! (Greedy Algorithm Series 1)
- Greedy Algorithm: 122. Best time to buy and sell stocks II
- Greedy Algorithm: 55. Jump Game
- Greedy Algorithm: 45. Jump Game II
- Greedy algorithm: Maximized array sum after 1005.K negations
- Summary of this week! (Greedy Algorithm Series 2)
- Greedy algorithm: 134. Gas station
- Greedy algorithm: 135. Distribute candy
- Greedy algorithm: 860. Lemonade change
- Greedy algorithm: 406. Reconstruct the queue based on height
- Summary of this week! (Greedy Algorithm Series 3)
- Greedy Algorithm: 406. Reconstruct Queue Based on Height (Sequel)
- Greedy Algorithm: 452. Detonate the balloon with the minimum number of arrows
- Greedy algorithm: 435. No overlapping intervals
- Greedy algorithm: 763. Divide letter intervals
- Greedy algorithm: 56. Merge intervals
- Summary of this week! (Greedy Algorithm Series 4)
- Greedy Algorithm: 738. Monotonically increasing numbers
- Greedy Algorithm: 968. Monitor Binary Tree
- Greedy Algorithm: Summary! (Every summary must be classic)
dynamic programming
The topic of dynamic programming has already started, there is no time to explain, friends, get on the bus and don’t fall behind!
- What you should know about dynamic programming!
- Dynamic programming: 509. Fibonacci numbers
- Dynamic Programming: 70. Climbing Stairs
- Dynamic programming: 746. Climb stairs with minimum cost
- Summary of this week! (Dynamic Planning Series 1)
- Dynamic programming: 62. Different paths
- Dynamic Programming: 63. Different Paths II
- Dynamic Programming: 343. Integer Split
- Dynamic Programming: 96. Different Binary Search Trees
- Summary of this week! (Dynamic Programming Series 2)
Backpack Problem Series:
- Dynamic programming: 01 Backpack theoretical basis
- Dynamic programming: 01 Backpack theoretical basis (rolling array)
- Dynamic Programming: 416. Partitioning Equisum Subsets
- Dynamic Programming: 1049. The Weight of the Last Stone II
- Summary of this week! (Dynamic Planning Series 3)
- Dynamic Programming: 494. Goal and
- Dynamic Programming: 474. Ones and Zeros
- Dynamic Programming: Complete Backpack Summary
- Dynamic Programming: 518. Change Exchange II
- Summary of this week! (Dynamic Programming Series 4)
- Dynamic Programming: 377. Combinatorial Sum IV
- Dynamic Programming: 70. Stair Climbing (Full Backpacking Version)
- Dynamic programming: 322. Change exchange
- Dynamic Programming: 279. Perfect Square Numbers
- Summary of this week! (Dynamic Programming Series 5)
- Dynamic Programming: 139. Word Splitting
- Dynamic Programming: Theoretical Basis of Multiple Knapsacks
- Summary of Backpack Problem
Robbery series:
- Dynamic Programming: 198. Robbery
- Dynamic Programming: 213. Robbery II
- Dynamic Programming: 337. Robbery III
Stock series:
- Dynamic Programming: 121. Best time to buy and sell stocks
- Dynamic Programming: Summary of this Week (Series 6)
- Dynamic Programming: 122. Best times to buy and sell stocks II
- Dynamic Programming: 123. Best time to buy and sell stocks III
- Dynamic Programming: 188. Best time to buy and sell stocks IV
- Dynamic programming: 309. The best time to buy and sell stocks includes the freezing period
- Dynamic Programming: Summary of this Week (Series 7)
- Dynamic programming: 714. The best time to buy and sell stocks including handling fees
- Dynamic Programming: Summary of Stock Series
Subsequence series:
- Dynamic programming: 300. Longest increasing subsequence
- Dynamic Programming: 674. Longest Continuous Increasing Sequence
- Dynamic Programming: 718. Longest Repeating Subarray
- Dynamic programming: 1143. Longest common subsequence
- Dynamic programming: 1035. Disjoint lines
- Dynamic programming: 53. Maximum subsequence sum
- Dynamic programming: 392. Determining subsequences
- Dynamic Programming: 115. Different subsequences
- Dynamic programming: 583. Deletion operation of two strings
- Dynamic Programming: 72. Edit Distance
- Edit distance summary
- Dynamic programming: 647. Palindrome substring
- Dynamic programming: 516. Longest palindrome subsequence
- Summary of dynamic programming
monotonic stack
- Monotone stack: 739. Daily temperature
- Monotone stack: 496. Next larger element I
- Monotonic stack: 503. Next larger element II
- Monotone stack: 42. Catching rainwater
- Monotonic stack: 84. Largest rectangle in histogram
graph theory
Graph theory officially released
- Graph theory: theoretical foundations
- Graph Theory: Theoretical Basis of Depth-First Search
- Graph theory: all reachable paths
- Graph Theory: Theoretical Basis of Breadth-First Search
- Graph Theory: Number of Islands. Deep Search Version
- Graph theory: Number of islands. Guangsou version
- Graph Theory: Maximum Area of an Island
- Graph theory: Total area of the island
- Graph Theory: The Sunken Island
- Graph theory: water flow problem
- Graph Theory: Building the Largest Island
- Graph Theory: String Solitaire
- Graph Theory: Complete Reachability of Directed Graphs
- Graph Theory: Perimeter of an Island
- Graph theory: basics of union search theory
- Graph Theory: Finding Paths to Existence
- Graph Theory: Redundant Connections
- Graph Theory: Redundant Connections II
- Graph theory: prim of minimum spanning tree
- Graph theory: kruskal of minimum spanning tree
- Graph Theory: Topological Sorting
- Graph theory: dijkstra (naive version)
- Graph theory: dijkstra (heap optimized version)
- Graph theory: Bellman_ford algorithm
- Graph Theory: Bellman_ford Queue Optimization Algorithm (aka SPFA)
- Graph theory: Bellman_ford’s judgment negative weight loop
- Graph theory: Bellman_ford's single source finite shortest path
- Graph Theory: Floyd’s Algorithm
- Graph theory: A* algorithm
- Graph Theory: Summary of Shortest Path Algorithm
- Graph Theory: Summary of Graph Theory
(continuously updated....)
Top ten ranking
number theory
Advanced data structure classic questions
- And search the collection
- minimum spanning tree
- Segment tree
- tree array
- dictionary tree
Massive data processing
Supplementary questions
The above questions are the top priority. You must study them at least twice to fully understand them. If you are proficient in the above questions and are still looking for other questions to practice, you can study the following questions again:
These questions are very good, but some of them are similar to the question brushing guide, and some of the problem solutions will be supplemented later, so I have not included them in the question brushing guide. I will improve some of the problem solutions in the future and then incorporate them into the problem solving strategy.
array
- 1365.How many numbers are there that are smaller than the current number?
- 941. Valid mountain array (double pointer)
- 1207. Classic application of unique array of occurrences in hashing
- 283. Move zero [array] [double pointer]
- 189. Rotate array
- 724.Finding the center index of an array
- 34. Find the first and last position of an element in a sorted array (bisection method)
- 922. Sort Array by Odd and Even II
- 35.Search for insertion position
linked list
- 24. Exchange nodes in the linked list pairwise
- 234. Palindrome linked list
- 143. Rearrange the linked list [array] [two-way queue] [directly operate the linked list]
- 141. Circular linked list
- 160. Intersected linked lists
Hash table
- 205. Isomorphic strings: [Application of hash table]
string
- 925. Long press to simulate matching
- 0844. Compare strings containing backspace [stack simulation] [double pointers with better space]
Binary tree
- 129. Find the sum of numbers from root to leaf nodes
- 1382. Convert the binary search tree to balance and construct a balanced binary search tree
- 100. The same tree has the same idea as 101. Symmetric binary tree
- 116. Fill the next right node pointer of each node
Backtracking algorithm
greedy
- 649.Dota2 Senate is difficult
- 1221. Splitting balanced characters is simple and greedy
dynamic programming
- 5. The longest palindrome substring is almost the same as the 647. palindrome substring.
- 132. Split palindrome string II is very similar to 647. Palindrome substring and 5. Longest palindrome substring
- 673.The number of longest increasing subsequences
graph theory
- 463.Perimeter of island (simulation)
- 841. Keys and rooms [directed graph] dfs, bfs can be used
- 127.Word Solitaire Guangsou
And search the collection
- 684.Redundant connection [Basic questions on combined search]
- 685. Redundant Connection II [Application of Union Lookup]
simulation
- 657.Can the robot return to the origin?
- 31.Next arrangement
Bit operations
- 1356. Sort according to the number of 1's in digital binary system
algorithm template
Various basic algorithm templates
Contributor
Click here to view all contributors to LeetCode-Master. Thanks to them for supplementing other language versions of LeetCode-Master so that more readers can benefit from this project.
Star Trends
About the author
Hello everyone, I am programmer Carl, a senior fellow at Harbin Institute of Technology and the author of "Code Captions". I have been engaged in the research and development of underlying back-end technologies at Tencent and Baidu.
PDF download
Add the following corporate WeChat account and the PDF version will be automatically sent to everyone. You can also choose whether to join the question answering group.
Remember to make a note when adding WeChat. If you are already working, note: name-city-position. If a student, please note: name-school-grade. Note: If you don’t introduce yourself, you won’t be able to pass.