React コンポーネント間の相互呼び出しでは、呼び出し元を親コンポーネント、呼び出し先を子コンポーネントと呼びます。親コンポーネントと子コンポーネント間で値を渡すことができます。 1. 親コンポーネントが子コンポーネントに値を渡すとき、渡される値はまず子コンポーネントに渡され、次に子コンポーネント内で props が使用されます。親コンポーネントから渡された値を受け取る; 2. 子コンポーネント 親コンポーネントに値を渡すときは、トリガーメソッドを通じて親コンポーネントに値を渡す必要があります。
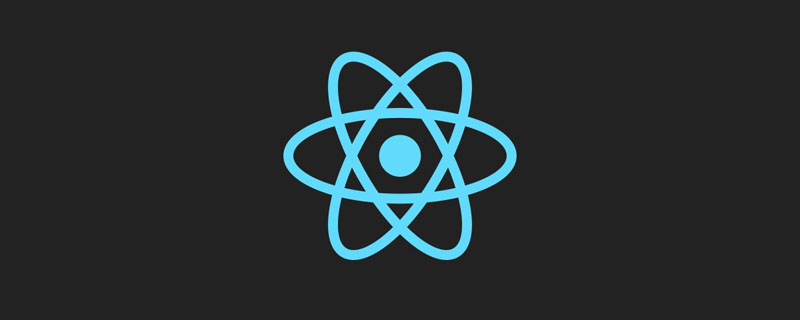
このチュートリアルの動作環境: Windows7 システム、react18 バージョン、Dell G3 コンピューター。
1. React のコンポーネント
反応コンポーネントは、独自に定義された非 HTML タグです。反応コンポーネントの最初の文字は大文字であることが規定されています。
クラス App はコンポーネントを拡張します{}<App />
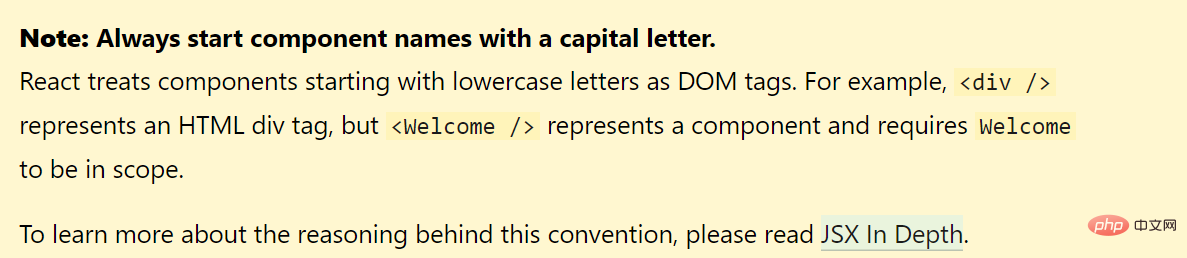
2. 親子コンポーネント
コンポーネントが相互に呼び出す場合、呼び出し元は親コンポーネントと呼ばれ、呼び出し先は子コンポーネントと呼ばれます。
import React from 'react';import Children from './Children';class Up extends React.Component {constructor(props){ super(props); this.state = { } } render(){ console.log("render "); return( <div> up <Children /> </div> ) }}export デフォルト Up;import React from 'react';class Children extends React.Component{ constructionor(props){ super(props); this. state = { } } render(){ return ( <div> Children </div> ) }}デフォルトのChildrenをエクスポートします。
3. 親コンポーネントが子コンポーネントに値を渡す
親コンポーネントは、props を使用して子コンポーネントに値を渡します。親コンポーネントが子コンポーネントに値を渡す場合、まず渡される値が子コンポーネントに渡され、次に子コンポーネントでは props を使用して親コンポーネントから渡された値を受け取ります。
親コンポーネントは、子コンポーネントを呼び出すときにプロパティを定義します。
<Children msg="親コンポーネントが値を子コンポーネントに渡します" />
この値 msg はサブコンポーネントの props 属性にバインドされ、サブコンポーネントは直接使用できます。
this.props.msg
親コンポーネントは値とメソッドをコンポーネントに渡すことができ、さらにはそれ自体を子コンポーネントに渡すこともできます。
3.1 値の受け渡し
import React from 'react';import Children from './Children';class Up extends React.Component {constructor(props){ super(props); this.state = { } } render(){ console.log("render "); return( <div> up <Children msg="親コンポーネントが子コンポーネントに値を渡します" /> </div> ) }}デフォルトのエクスポート Up;React からのインポート 'react';class Children extends React.Component{ コンストラクター(props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> {this.props.msg} </div> ) }}デフォルトの子をエクスポートします。
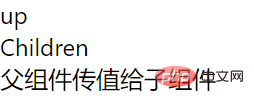
3.2 送信方法
import React from 'react';import Children from './Children';class Up extends React.Component { constructionor(props){ super(props); run = () => { console.log ("親コンポーネントの実行メソッド"); } render(){ console.log("render"); return( <div> up <Children run={this.run} /> </div> ) }}export デフォルト Up ;import React from 'react';class Children extends React.Component{ constructionor(props){ super(props); this.state = { } } run = () => { this.props.run(); ){ return ( <div> 子 <br /> <button onClick={this.run}>実行</button> </div> ) }}デフォルトの子をエクスポートします。
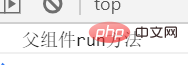
3.3 親コンポーネントを子コンポーネントに渡す
import React from 'react';import Children from './Children';class Up extends React.Component { constructionor(props){ super(props); run = () => { console.log ("親コンポーネントの実行メソッド"); } render(){ console.log("render"); return( <div> up <Children msg={this}/> </div> ) }}export デフォルト Up;import React from 'react';class Children extends React.Component{ constructionor(props){ super(props); this.state = { } } run = () => { console.log(this.props.msg); (){ return ( <div> 子 <br /> <button onClick={this.run}>実行</button> </div> ) }}デフォルトの子をエクスポートします。
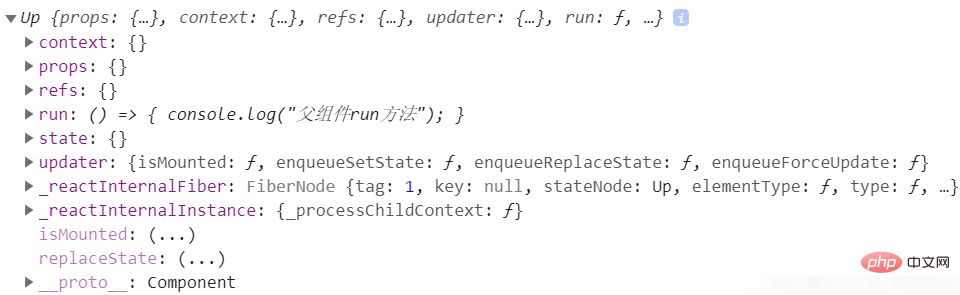
4. サブコンポーネントが親コンポーネントに値を渡す
子コンポーネントは、トリガー メソッドを通じて値を親コンポーネントに渡します。
import React from 'react';import Children from './Children';class Up extends React.Component {constructor(props){ super(props); this.state = { } } getChildrenData = (data) => { console. log(data); } render(){ console.log("render"); return( <div> up <Children upFun={this.getChildrenData}/> </div> ) }}export デフォルト Up;import 'react';class Children extends React.Component{ constructionor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> <button onClick={() = > {this.props.upFun("子コンポーネント データ")}}>Run</button> </div> ) }}デフォルトの子をエクスポートします。
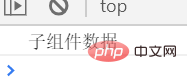
5. 親コンポーネントの参照を通じてサブコンポーネントのプロパティとメソッドを取得する
import React from 'react';import Children from './Children';class Up extends React.Component {constructor(props){ super(props); this.state = { } } clickButton = () => { console.log (this.refs.children); } render(){ console.log("render"); return( <div> up <Children ref="children" msg="test"/> <button onClick={this.clickButton }>click</button> </div> ) }}export デフォルト Up;``````jsimport React from 'react';class Children extends React.Component{ constructionor(props){ super(props); state = { title: "サブコンポーネント" } } runChildren = () => { } render(){ return ( <div> Children <br /> </div> ) }}export デフォルトの Children;````