React 구성 요소 간의 상호 호출에서 호출자를 상위 구성 요소라고 하고 호출 수신자를 하위 구성 요소라고 합니다. 부모 컴포넌트와 자식 컴포넌트 사이에 값을 전달할 수 있습니다. 1. 부모 컴포넌트가 자식 컴포넌트에 값을 전달하면 전달할 값이 먼저 자식 컴포넌트에 전달되고, 그 다음 자식 컴포넌트에서는 props를 사용하여 2. 자식 컴포넌트 부모 컴포넌트에 값을 전달할 때, 트리거 메소드를 통해 부모 컴포넌트에 값을 전달해야 합니다.
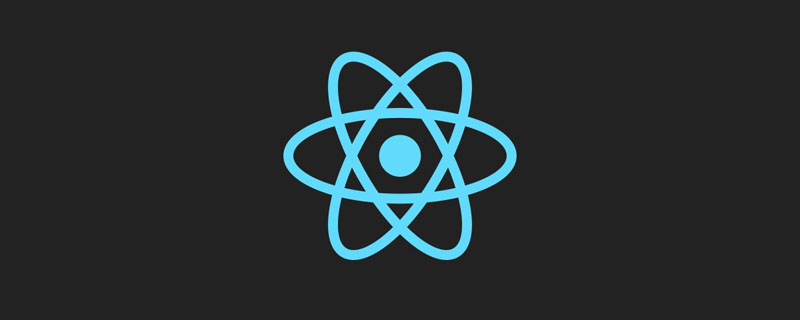
이 튜토리얼의 운영 환경: Windows7 시스템, React18 버전, Dell G3 컴퓨터.
1. React의 구성 요소
반응 구성 요소는 자체 정의된 HTML이 아닌 태그입니다. 반응 구성 요소의 첫 글자는 대문자로 표시됩니다.
클래스 App은 구성 요소를 확장합니다{}<App />
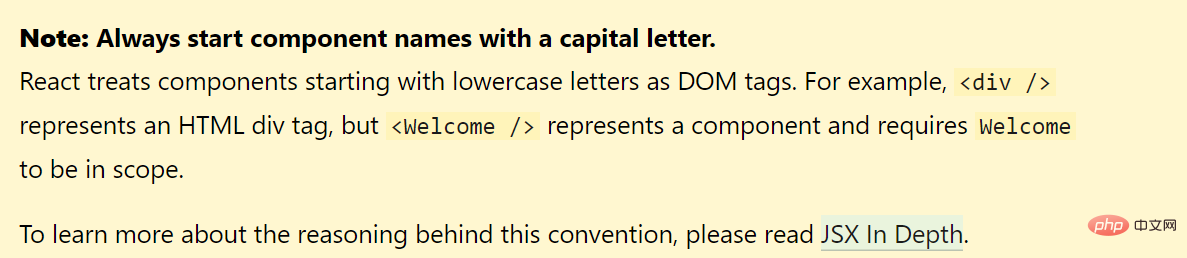
2. 상위-하위 구성요소
구성 요소가 서로 호출할 때 호출자를 상위 구성 요소라고 하고 호출 수신자를 하위 구성 요소라고 합니다.
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props) this.state = { } } render(){ console.log("render "); return( <div> up <Children /> </div> ) }}기본 내보내기 Up;'react'에서 React 가져오기;class Children은 React.Component를 확장합니다. constructor(props){ super(props); this. state = { } } render(){ return ( <div> Children </div> ) }}기본 Children 내보내기;
3. 상위 구성 요소는 하위 구성 요소에 값을 전달합니다.
상위 컴포넌트는 props를 사용하여 하위 컴포넌트에 값을 전달합니다. 부모 컴포넌트가 자식 컴포넌트에 값을 전달할 때 전달할 값이 먼저 자식 컴포넌트에 전달되고, 그 다음 자식 컴포넌트에서는 props를 사용하여 부모 컴포넌트가 전달한 값을 받습니다.
상위 구성 요소는 하위 구성 요소를 호출할 때 속성을 정의합니다.
<Children msg="상위 구성 요소가 하위 구성 요소에 값을 전달합니다." />
이 값 msg는 하위 구성 요소의 props 속성에 바인딩되며 하위 구성 요소를 직접 사용할 수 있습니다.
this.props.msg
상위 구성 요소는 값과 메서드를 구성 요소에 전달할 수 있으며, 심지어 하위 구성 요소에도 자신을 전달할 수도 있습니다.
3.1 전달 값
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props) this.state = { } } render(){ console.log("render "); return( <div> up <Children msg="상위 구성 요소가 하위 구성 요소에 값을 전달합니다" /> </div> ) }}export default Up;import React from 'react';class Children은 React.Component를 확장합니다{ 생성자 (props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> {this.props.msg} </div> ) }}기본 Children 내보내기;
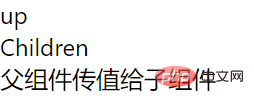
3.2 전송방식
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props) this.state = { } } run = () => { console.log ("상위 구성 요소 실행 방법"); } render(){ console.log("render"); return( <div> up <Children run={this.run} /> </div> ) }}기본값 내보내기 ;'react'에서 React 가져오기;class Children은 React.Component{ constructor(props){ super(props); this.state = { } } run = () => { this.props.run(); ){ return ( <div> 하위 <br /> <button onClick={this.run}>실행</button> </div> ) }}기본 하위 내보내기;
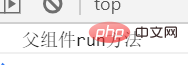
3.3 상위 구성요소를 하위 구성요소에 전달
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props) this.state = { } } run = () => { console.log ("상위 구성 요소 실행 방법"); } render(){ console.log("render"); return( <div> up <Children msg={this}/> </div> ) }}export default Up;import React from 'react';class Children은 React.Component{ constructor(props){ super(props); this.state = { } } run = () => { console.log(this.props.msg); (){ return ( <div> 하위 <br /> <button onClick={this.run}>실행</button> </div> ) }}기본 하위 내보내기;
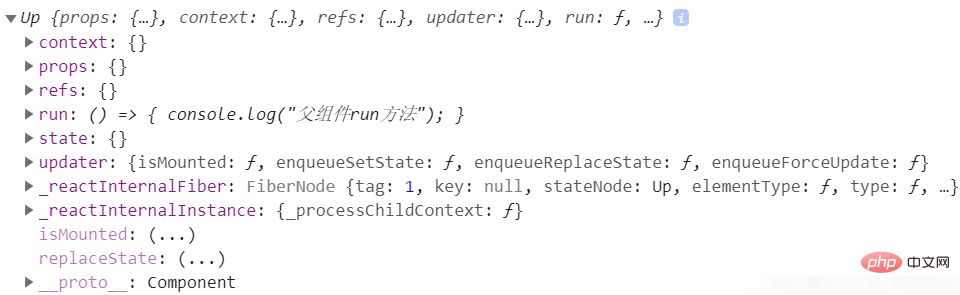
4. 하위 구성 요소는 상위 구성 요소에 값을 전달합니다.
하위 구성 요소는 트리거 메서드를 통해 상위 구성 요소에 값을 전달합니다.
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props); this.state = { } } getChildrenData = (data) => { console. log(data); } render(){ console.log("render"); return( <div> up <Children upFun={this.getChildrenData}/> </div> ) }}기본값 내보내기 Up;import React from 'react';class Children은 React.Component를 확장합니다. constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> <button onClick={() = > {this.props.upFun("child component data")}}>실행</button> </div> ) }}기본 자식 내보내기;
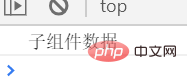
5. 상위 구성 요소의 참조를 통해 하위 구성 요소 속성 및 메서드 얻기
import React from 'react';import Children from './Children';class Up 확장 React.Component { constructor(props){ super(props) this.state = { } } clickButton = () => { console.log (this.refs.children); } render(){ console.log("render"); return( <div> up <Children ref="children" msg="test"/> <button onClick={this.clickButton }>클릭</button> </div> ) }}export default Up;``````jsimport 'react'의 React;class Children은 React.Component{ constructor(props){ super(props) this를 확장합니다. state = { title: "하위 구성 요소" } } runChildren = () => { } render(){ return ( <div> Children <br /> </div> ) }}기본 Children 내보내기;````