In the mutual calls between react components, the caller is called the parent component and the callee is called the child component. Values can be passed between parent and child components: 1. When a parent component passes a value to a child component, the value to be passed is first passed to the child component, and then in the child component, props are used to receive the value passed by the parent component; 2. Child component When passing values to the parent component, you need to pass them to the parent component through the trigger method.
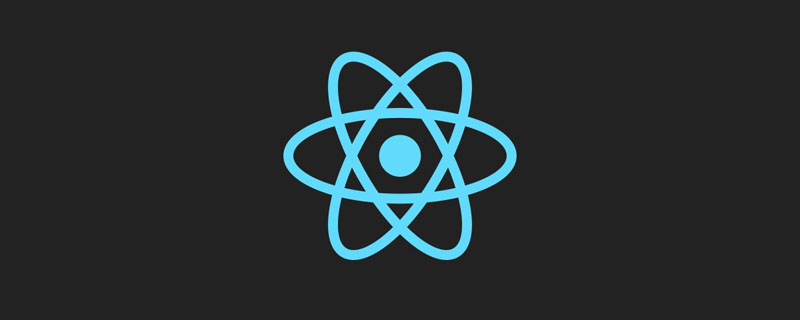
The operating environment of this tutorial: Windows7 system, react18 version, Dell G3 computer.
1. Components in React
The react component is a self-defined non-html tag. It is stipulated that the first letter of the react component is capitalized:
class App extends Component{}<App />
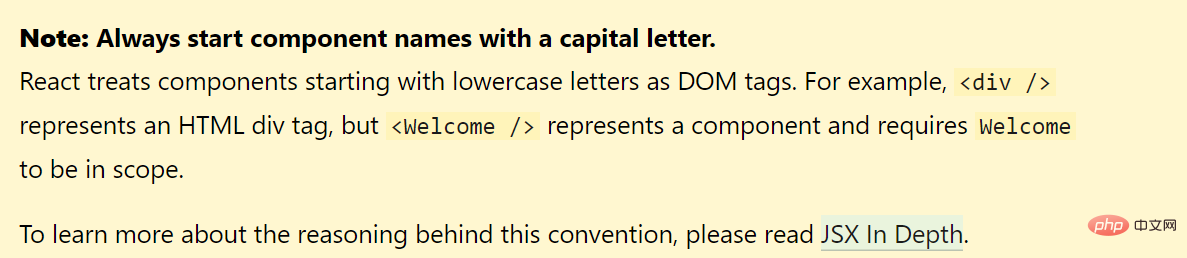
2. Parent-child components
When components call each other, the caller is called the parent component and the callee is called the child component:
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render "); return( <div> up <Children /> </div> ) }}export default Up;import React from 'react';class Children extends React.Component{ constructor(props){ super(props); this. state = { } } render(){ return ( <div> Children </div> ) }}export default Children;
3. Parent component passes value to child component
Parent components pass values to child components using props. When a parent component passes a value to a child component, the value to be passed is first passed to the child component, and then in the child component, props are used to receive the value passed by the parent component.
The parent component defines a property when calling the child component:
<Children msg="Parent component passes value to child component" />
This value msg will be bound to the props attribute of the subcomponent, and the subcomponent can be used directly:
this.props.msg
Parent components can pass values and methods to components, and can even pass themselves to child components.
3.1 Passing value
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render "); return( <div> up <Children msg="Parent component passes value to child component" /> </div> ) }}export default Up;import React from 'react';class Children extends React.Component{ constructor (props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> {this.props.msg} </div> ) }}export default Children;
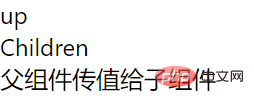
3.2 Transmission method
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log ("parent component run method"); } render(){ console.log("render"); return( <div> up <Children run={this.run} /> </div> ) }}export default Up ;import React from 'react';class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { this.props.run(); } render( ){ return ( <div> Children <br /> <button onClick={this.run}>Run</button> </div> ) }}export default Children;
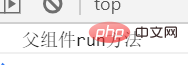
3.3 Pass parent component to child component
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log ("parent component run method"); } render(){ console.log("render"); return( <div> up <Children msg={this}/> </div> ) }}export default Up;import React from 'react';class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { console.log(this.props.msg); } render (){ return ( <div> Children <br /> <button onClick={this.run}>Run</button> </div> ) }}export default Children;
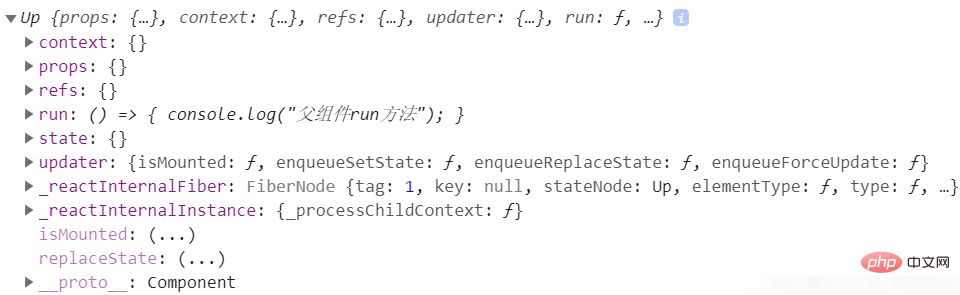
4. Subcomponent passes value to parent component
The child component passes the value to the parent component through the trigger method.
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } getChildrenData = (data) => { console. log(data); } render(){ console.log("render"); return( <div> up <Children upFun={this.getChildrenData}/> </div> ) }}export default Up;import React from 'react';class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br /> <button onClick={() = > {this.props.upFun("child component data")}}>Run</button> </div> ) }}export default Children;
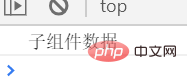
5. Obtain sub-component properties and methods through refs in the parent component
import React from 'react';import Children from './Children';class Up extends React.Component { constructor(props){ super(props); this.state = { } } clickButton = () => { console.log (this.refs.children); } render(){ console.log("render"); return( <div> up <Children ref="children" msg="test"/> <button onClick={this.clickButton }>click</button> </div> ) }}export default Up;``````jsimport React from 'react';class Children extends React.Component{ constructor(props){ super(props); this. state = { title: "Subcomponent" } } runChildren = () => { } render(){ return ( <div> Children <br /> </div> ) }}export default Children;````